Create a FlexSlider Gallery Using Attachment Images in WordPress without a Plugin
Posted on April 22nd, 2014 in Tutorials
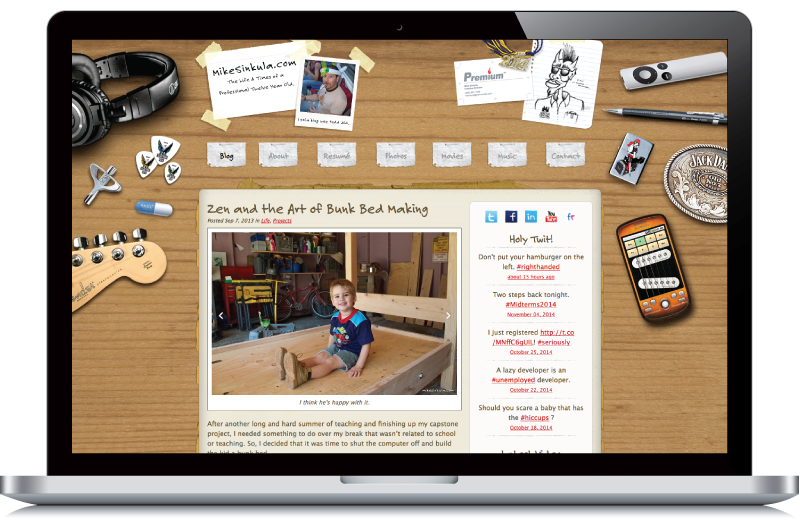
Have you ever wanted to automatically pull multiple image attachments from a WordPress posting and present them as a Flexslider gallery without needing to insert short-code or use a plugin? Yes? Then this tutorial is for you!
The Challenge
For some time now, I have been a fan of using Flexslider by WooThemes as an image gallery in my websites. I have even written up an exercise on how to implement it into a static site for my Human-Centered Design & Engineering students at the University of Washington.
See Also: Create an Image Slider with jQuery | HCDE598 | Premium Design Works
But, since I don’t really run any static sites any longer, I had to figure out how to integrate it into my WordPress sites.
In the past, I had used various gallery plugins that used shortcode:
The issue with this was that I had to write said shortcode and it’s attributes into each posting which is relatively inefficient. Basically, I did not want have to copy and paste shortcode from one posting to the next any longer.
See Also: Shortcode API | WordPress Codex
Instead, what I wanted was to have a gallery automatically render the images that I uploaded to each of the postings without any hassle. I also knew that I wanted this gallery to display at the top of all my postings.
Since I was already armed with Flexslider by WooThemes, the question then became, “How do I integrate this without using a plugin?”
Integrating Flexslider by itself is relatively easy.
First, you need to download the package and put the files in the necessary place:
Once you have downloaded the package and placed the files in the correct place, you will need to hook the files up.
First, link to the stylesheet in your header.php file:
<link rel="stylesheet" href="<?php bloginfo('template_directory'); ?>/flexslider.css" type="text/css" media="all" />
Second, link to the JavaScript in your header.php file:
<script src="<?php bloginfo('template_directory'); ?>/scripts/jquery.flexslider.js"></script>
Note: Don’t forget to link to the jQuery Library first!
Lastly, hook it up with the JQuery function in your header.php file:
<script type="text/javascript"> $(window).load(function(){ $('.flexslider').flexslider(); }) </script>
But, when it comes to pulling the images from a posting without using a plugin is where the challenge was for me.
The Solution
This is where I needed to figure out a way to pull the images from a WordPress posting and write them into the markup that FlexSlider required.
The function that I wrote was, to me, both elegant and simple.
You can see this function in my functions.php file:
<?php function add_flexslider() { // display attachment images as a flexslider gallery on single posting global $post; // don't forget to make this a global variable inside your function $attachments = get_children(array('post_parent' => $post->ID, 'order' => 'ASC', 'orderby' => 'menu_order', 'post_type' => 'attachment', 'post_mime_type' => 'image', )); if ($attachments) { // if there are images attached to posting, start the flexslider markup echo '<div class="flexslider">'; echo '<ul class="slides">'; foreach ( $attachments as $attachment_id => $attachment ) { // create the list items for images with captions echo '<li>'; echo wp_get_attachment_image($attachment_id, 'large'); echo '<p>'; echo get_post_field('post_excerpt', $attachment->ID); echo '</p>'; echo '</li>'; } echo '</ul>'; echo '</div>'; } // end see if images } // end add flexslider ?>
This is where I first needed to pull the array of data from WordPress using the get_children() function that can retrieve the attachments to a posting. I then needed to re-key the array to set my parameters of the gallery and declare it as the $attachments variable.
From there, it was a matter of writing a conditional statement to see if there were images attached to the posting. If there were, write the FlexSlider markup and use a foreach loop to call both the image using the wp_get_attachment_image() function and it’s caption using the get_post_field() function.
Once that function was written, I just needed to place the call to it within my single.php file:
<?php add_flexslider(); ?>
Now, every time I create a new posting and add images to it, it will automatically display as a FlexSlider gallery!
Hi Mike,
I have used your code exactly and I cannot to get it to work—I suspect, perhaps, because the images I am trying to pull are inserted from hyperlinks. Please see my full question on wordpress.org for more details:
http://wordpress.org/support/topic/pull-all-postpage-images-for-slider?replies=1
Would you be willing to help a brother out? Many thanks in advance!
Hi
Thank you very much to share this.
I need this slider with thumbnails and nav.
http://flexslider.woothemes.com/thumbnail-controlnav.html
Please add this in this tutorial.
For slider with thumbnails use this JS:
And for function.php use this code:
Hope it helps!
Exactly what I’m looking for, but images aren’t loading. Does the code for functions.php need to be changed for a custom post type?
Thank you!
As long as the images are “attached” to the custom post type, I don’t think it should matter. Remember, hot-linked images from other sites will not work.
There is a problem. the $attachment var gives:
– Undefined variable
– Trying to get property of non-object
just in thi line:
Ah ok… the problem is that you are trying to ghet the caption from a var that you are just asigning value to so in the time of doing it it’s empty.
You are actually right. You don’t need
So, it’s been removed from the tutorial.
Hi, I love this function. thanks for sharing. The only thing I couldn’t find is a way to add a link to a large version of the image when you click over them.
Can you help me with it?
According to https://codex.wordpress.org/Function_Reference/wp_get_attachment_image_src you would do something like this:
'; echo get_post_field('post_excerpt', $attachment->ID); echo '
'; echo 'Thank you Mike. Works like charm
hi there, im almost there, but the images wont show – they have loaded in the source code but something is preventing the jquery showing them again
please help – see link,
regards craig
Damn it!
I have a new problem here.
In standard post. this function worked very well.
But… I decided to create a custom post type because I am manipulating products, so I created a custom post type called “product” and the function didn’t work anymore.
Any suggestion?
Mike, I’ve got my error. It was in other place (it was in my function to show custom post types in category template.)
In case somebody use custom post types and want to show attachment images with flexislider in category template.
I recommend this function explained in this article.
http://premium.wpmudev.org/blog/add-custom-post-types-to-tags-and-categories-in-wordpress/?dsh=1&utm_expid=3606929-40.lszTaIEzTbifDhvhVdd39A.1&utm_referrer=https%3A%2F%2Fwww.google.com.ar%2F
Everything will works better.
im having a problem, the code is working some how but its calling an image in media folder not the image attachment field from the custom post type, any idea ?
A rolling stone is worth two in the bush, thanks to this arieclt.
Sosterà, I’m not so expert in wordpress and php so what do you mean for script folder and root folder?
Thank you
Root folder is the root folder of WordPress, where wp-config.php file is located. Scripts folder you can create by yourself or maybe it is already there, and it is usually located at “\wp-content\themes\[Your-Theme-Name]\scripts”.
Sorry Claudio, Mike just corrected me, in this case, the ‘root folder’ is actually the Theme’s folder. For instance
\wp-content\themes\[Your-Theme-Name]
.Hello, please help, the images are showing in my source code but not on the front end. Exaclty as @craig simpson’s issue above. What is causing this?
If anyone else has this problem I found a workaround:
In my Chrome Inspector, under Console Tab, it output 2 errors:
1) Uncaught ReferenceError: jQuery is not defined
2) Uncaught TypeError: $ is not a function
The 1st error is fixed by placing the script AFTER any wp_head(); calls. This makes sure no conflicts with previous jQuery calls.
The 2nd error is fixed by using the JQuery’s ‘noConflict’ mode, because the string ($) is laready “taken”, so to speak:
var jq = jQuery.noConflict(); // I declared "jq" instead of "$"
and then replace “$” further in the code:
jq(window).load(function(){
jq('.flexslider').flexslider({
animation: "fade",
slideshow: false,
});
})
Mike, I would like to know is it safe to leave the code like this in WordPress environment? I wouldn’t want to leave it like his if it will create new complications further along (if it is a hack, and not a clean solution).
HI,
very nice code for gallery. I have problem with this code it grabs all the images for slider. I would like to control what image should be in slider and what should not be. How can i achieve this? I have slider and I have a product picture I don’t want to add this product picture into slider.
I will appreciate your help.
thanks in advance
When inserted, the needles keep in place for several minutes, which
normally does not lead to substantially pain.
Sorry, silly question where do you put the js code for the slide with nav?
Save as file name / type? In scripts folder?
Sorry bit of a noob.
Many thanks.
Its cool I found where, not working but I found it lol
Great tutorial!
I hope you can help me figure out how to add a post title as the navigational link?